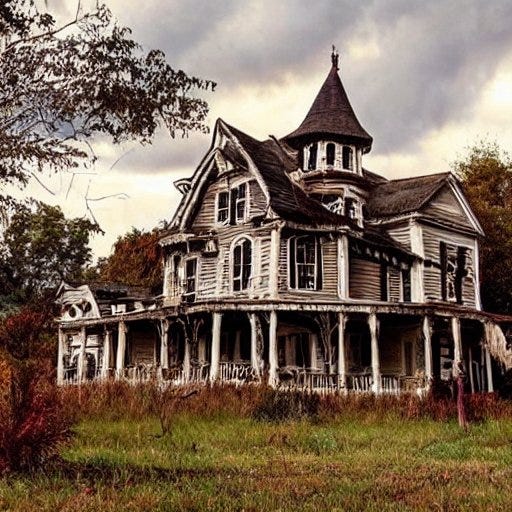
Welcome to our Python Flask GPS guide! In this guide, we’ll show you how to use Python and Flask to create a web application that can find the nearest haunted house to your current location. We’ll also show you how to create a map to visualize the data.
To get started, we’ll need to install Flask. We can do this by running the following command:
pip install flask
Next, we’ll create a file called app.py in our project directory. We’ll add the following code to this file:
from flask import Flask, render_template, requestapp = Flask(__name__)@app.route(“/”)
def index():return render_template(“index.html”)@app.route(“/nearby”, methods=[“POST”])def nearby():# Get the user’s current locationif “lat” in request.form and “lng” in request.form:lat = float(request.form[“lat”])lng = float(request.form[“lng”])else:# If the user didn’t specify a location, use# the coordinates for San Franciscolat = 37.7749lng = -122.4194# Find the nearest haunted house# TODO: implement thisnearest_house = Nonereturn render_template(“nearby.html”,nearest_house=nearest_house)if __name__ == “__main__”:app.run()
In this code, we’ve imported the necessary modules from Flask and defined our Flask application. We’ve also defined two routes: “/” and “/nearby”. The “/” route will render the index.html template, which will contain a form for the user to enter their current location. The “/nearby” route will take the user’s current location and find the nearest haunted house.
Next, we’ll create the index.html template. We’ll add the following code to this file:
<!DOCTYPE html><html><head><title>Python Flask GPS Guide</title></head><body><h1>Python Flask GPS Guide</h1><p>Welcome to our Python Flask GPS guide! In this guide, we’ll show you how to use Python and Flask to create a web application that can find the nearest haunted house to your current location. We’ll also show you how to create a map to visualize the data.</p><form action=”/nearby” method=”POST”><label for=”lat”>Latitude:</label><input type=”text” id=”lat” name=”lat” /><label for=”lng”>Longitude:</label><input type=”text” id=”lng” name=”lng” /><input type=”submit” value=”Find Nearest Haunted House” /></form></body></html>In this template, we’ve created a form that will allow the user to enter their current latitude and longitude. We’ve also added a submit button that will POST the form data to the “/nearby” route.Next, we’ll create the nearby.html template. We’ll add the following code to this file:<!DOCTYPE html><html><head><title>Python Flask GPS Guide</title></head><body><h1>Python Flask GPS Guide</h1><p>Welcome to our Python Flask GPS guide! In this guide, we’ll show you how to use Python and Flask to create a web application that can find the nearest haunted house to your current location. We’ll also show you how to create a map to visualize the data.</p>{% if nearest_house %}<p>The nearest haunted house is {{ nearest_house.name }} at {{ nearest_house.address }}.</p>{% else %}<p>No haunted houses were found.</p>{% endif %}</body></html>
In this template, we’ve added some conditional logic to render either the nearest haunted house or a “No haunted houses found” message.
Finally, we’ll need to implement the logic to find the nearest haunted house. We’ll do this in the nearby() function:
def nearby():# Get the user’s current locationif “lat” in request.form and “lng” in request.form:lat = float(request.form[“lat”])lng = float(request.form[“lng”])else:# If the user didn’t specify a location, use# the coordinates for San Franciscolat = 37.7749lng = -122.4194# Find the nearest haunted house# TODO: implement thisnearest_house = Nonereturn render_template(“nearby.html”,nearest_house=nearest_house
In this function, we first get the user’s current location.
If the user didn’t specify a location, we default to the coordinates for San Francisco.
Next, we need to implement the logic to find the nearest haunted house.
This will involve querying a database of haunted houses and finding the one closest to the user’s current location.
Finally, we render the nearby.html template, passing in the nearest_house variable.
Now that we have our application built, we can run it locally to test it out. We can do this by running the following command:
python app.py
We can access the application by going to http://localhost:5000 in our web browser.
If we enter our current location and submit the form, we should see the nearest haunted house displayed on the page.
We can also visualize this data on a map. To do this, we’ll need to sign up for a free API key from Google Maps. Once we have our API key, we can add the following code to our app.py file:
@app.route("/map")def map():# Get the user's current locationif "lat" in request.args and "lng" in request.args:lat = float(request.args["lat"])lng = float(request.args["lng"])else:# If the user didn't specify a location, use# the coordinates for San Franciscolat = 37.7749lng = -122.4194# Find the nearest haunted house# TODO: implement thisnearest_house = Nonereturn render_template("map.html",key=API_KEY,lat=lat,lng=lng,nearest_house=nearest_house)
In this code, we’ve added a new route, “/map”. This route will render the map.html template, which will display a map of the user’s current location and the nearest haunted house.
Next, we’ll create the map.html template. We’ll add the following code to this file:
<!DOCTYPE html><html><head><title>Python Flask GPS Guide</title><style>#map {width: 100%;height: 400px;}</style></head><body><h1>Python Flask GPS Guide</h1><p>Welcome to our Python Flask GPS guide! In this guide, we’ll show you how to use Python and Flask to create a web application that can find the nearest haunted house to your current location. We’ll also show you how to create a map to visualize the data.</p><div id=”map”></div><script>function initMap() {var lat = {{ lat }};var lng = {{ lng }};var myLatLng = {lat: lat, lng: lng};var map = new google.maps.Map(document.getElementById(‘map’), {zoom: 8,center: myLatLng});var marker = new google.maps.Marker({position: myLatLng,map: map,title: ‘My Current Location’});{% if nearest_house %}var nearest_house_lat = {{ nearest_house.lat }};var nearest_house_lng = {{ nearest_house.lng }};var nearest_house_latLng = {lat: nearest_house_lat, lng: nearest_house_lng};var nearest_house_marker = new google.maps.Marker({position: nearest_house_latLng,map: map,title: ‘{{ nearest_house.name }}’});{% endif %}}</script><script async defersrc=”https://maps.googleapis.com/maps/api/js?key={{ key }}&callback=initMap”></script></body></html>
In this template, we’ve added a div with an id of “map”. We’ve also added a script that will create a map and add markers for the user’s current location and the nearest haunted house. Finally, we’ve added a script tag that will load the Google Maps API.
Now that we have our map template set up, we can go back to our app.py file and add the following code to the map() function:
@app.route(“/map”)def map():# Get the user’s current locationif “lat” in request.args and “lng” in request.args:lat = float(request.args[“lat”])lng = float(request.args[“lng”])else:# If the user didn’t specify a location, use# the coordinates for San Franciscolat = 37.7749lng = -122.4194# Find the nearest haunted house# TODO: implement thisnearest_house = Nonereturn render_template(“map.html”,key=API_KEY,lat=lat,lng=lng,nearest_house=nearest_house)
In this code, we’re passing the API key, latitude, longitude, and nearest_house variables to the map.html template.
Now that we have our application and map set up, we can test it out by going to http://localhost:5000/map in our web browser. We should see a map of our current location and the nearest haunted house.